What Is Excel VBA SendKeys?
In Excel VBA, SendKeys is a method that allows you to simulate keystrokes to interact with an active window or application. It can automate tasks by sending keyboard inputs to control various actions within Excel or other applications.
The SendKeys method takes a string parameter representing the keystrokes you want to send. Each character in the string represents a key or a combination of keys. For example, the string “Hello” will send the keys “H,” “e,” “l,” “l,” and “o” to the active window. We can also implement other keys like Enter, Backspace, Escape, etc.
Consider the example:
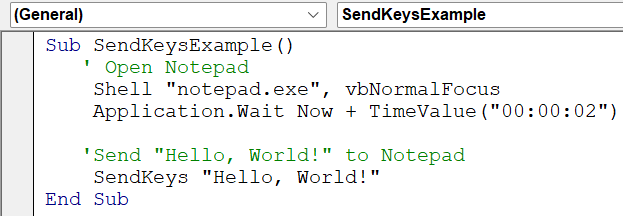
It will open the notepad file and print “Hello, World” in intervals of 2 seconds.
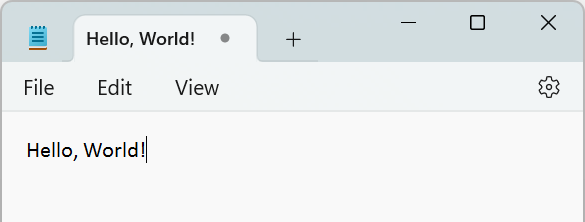
Table of contents
Key Takeaways
- SendKeys is used to send keystrokes to the active window or application.
- Each key is represented by one or more characters. These have a special meaning to SendKeys.
- Some applications or security configurations may block or restrict the use of SendKeys for automation. Make sure the target application allows the use of SendKeys.
- SendKeys can have varying results across different versions of Excel or operating systems. Test your code thoroughly and be prepared to handle any inconsistencies that may arise.
Excel VBA 5-in-1 Course Bundle – (30+ Hours of Expert-Led Training)
If you want to learn Excel and VBA professionally, then the Excel VBA 5-in-1 Course Bundle (30+ hours) is the perfect solution. Whether you’re a beginner or an experienced user, this bundle covers it all – from Basic Excel VBA to Event Automation, Error Handling, Email Automation, and real-world applications with downloadable templates.
Syntax
The syntax for VBA SendKeys is as shown below:
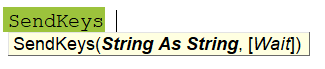
The SendKeys method accepts two optional arguments:
- string: This mandatory parameter specifies the keystrokes or key combinations you want to send. It can be a string literal or a variable containing the keystrokes. For example, “Hello” or myVariable.
- Wait: This is an optional parameter that specifies whether VBA should wait for the keystrokes to be processed by the active window before continuing with the code execution. It can take one of the following values:
- True or 1: VBA waits for the keystrokes to be processed (default behavior if omitted).
- False or 0: VBA does not wait and continues immediately after sending the keystrokes.
- Note that some special characters require special treatment when using SendKeys. For example, to send special characters like Enter or Tab, you can use the following representations in the string argument:
- Enter: {ENTER}
- Tab: {TAB}
- Backspace: {BACKSPACE}
- Escape: {ESC}
- It is only possible to implement VBA Sendkeys on keys, which can affect a document. Hence, VBA Sendkeys Numlock isn’t possible, not VBA send-keys Capslock.
How To Use Excel VBA SendKeys Method?
Consider an example where you implement VBA Sendkeys Enter in any place in the Excel VBA module.
We are trying to print VBA Sendkeys “Hello, World!” and “Open” in the following line. We must call VBA Sendkeys Enter to move the cursor to the next line.
Hence, a step-by-step guide is given below:
- Go to the “Developer” section in the toolbar and click the “Visual Basic” option. Now, the VBA Editor opens to add functions and sub-procedures. Then, click the “Insert” and “Module” buttons to create a new module or blank page.
- Initialize a sub-procedure to implement Excel VBA Sendkeys.
- Print “Hello, world!” where the cursor is present in VBA. For demonstration purposes, the cursor is in the Immediate tab.
The “True” value in this indicates that VBA will wait for a few seconds before deploying the keystroke. - Similarly, implement Excel VBA Sendkeys Enter along with the string “Open”. This will work as how the “Enter” key will typically work, move the cursor down, and print “Open.”
Code:
Sub SendKeysfunc()
SendKeys “Hello, World!”, True
SendKeys “{ENTER} Open”, False
End Sub - To run the code, press the run button or the F5 button. The output is printed in the Immediate tab.
Note: This will work anywhere the cursor is placed in the same VBA module. So, you can choose where you need strings to be printed in your module.
Examples
Let us look at a few examples of implementing VBA Sendkeys in our code.
Example #1
Consider an example where you must implement VBA Sendkeys Ctrl+C in a notepad. It can similarly be implemented using VBA Sendkeys Ctrl+V.
- Step 1: Declare a sub-procedure to implement VBA Sendkeys Ctrl+C

- Step 2: Open Notepad using the shell function in Excel VBA after a delay of 2 seconds.

- Step 3: Activate the Notepad function to perform the Excel VBA Sendkeys function.

- Step 4: Implement Ctrl+C in the newly opened notepad file and print “Ctrl+C is implemented”.

Code:
Sub SendKeysCtrlCExample()
Shell “notepad.exe”, vbNormalFocus
Application.Wait Now + TimeValue(“00:00:02”)
AppActivate “Notepad”
SendKeys “^c ctrl+c is implemented”
End Sub
- Step 5: Press F5 or the green arrow button to run the VBA code. The output is shown below:
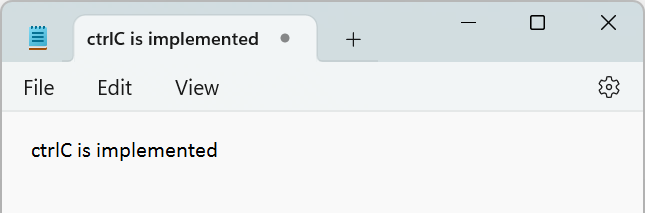
As observed, the space before “ctrl” is where Ctrl+C has been implemented. When no text is returned in the case of Ctrl+C, it leaves a blank space and then continues printing the rest of the sentence.
Example #2
Consider an example where you need to open a calculator and perform VBA Sendkey integers to the calculator directly. We can open the Windows built-in calculator to input some integers.
- Step 1: Declare a sub-procedure to open the Windows calculator.

- Step 2: Open Windows calculator with normal focus function with a delay of 1 second.

- Step 3: Activate the calculator application to implement the VBA Sendkeys function.

- Step 4: Using VBA Sendkeys print “123” on the calculator display.

Code:
Sub SendKeysNumericExample()
Shell “calc.exe”, vbNormalFocus
Application.Wait Now + TimeValue(“00:00:01”) ‘
AppActivate “Calculator”
SendKeys “123”
End Sub
- Step 5: Run the code using F5 or the Run button. The output is shown below.
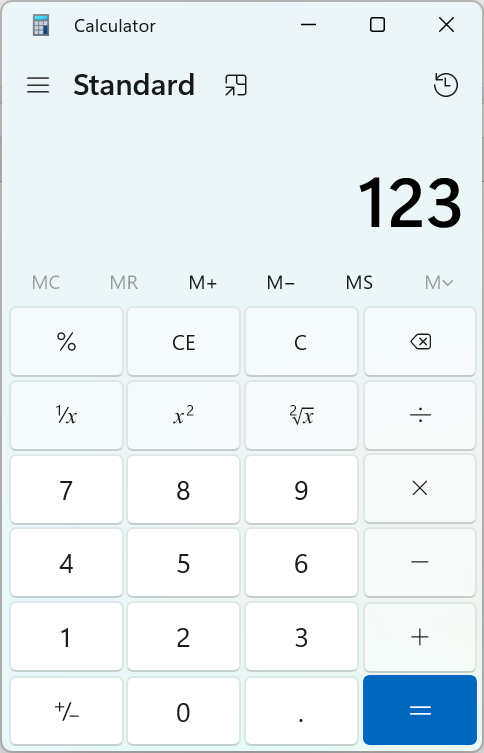
Important Things To Note
- Do use SendKeys sparingly and only when necessary, as they are a bit complex.
- Do use SendKeys where alternative methods are not available or feasible.
- Ensure the target window or application is active and ready to receive the keystrokes before using SendKeys.
- Use appropriate delays (Application.Wait) to allow sufficient time for the target application to respond to the keystrokes.Don’t rely solely on SendKeys for complex or critical automation tasks. Consider using the Excel object model or external libraries for more robust and controlled automation.
- Don’t use SendKeys for applications with built-in automation capabilities or available APIs.
- There is no consistent behavior across different Excel or operating systems versions when using SendKeys.
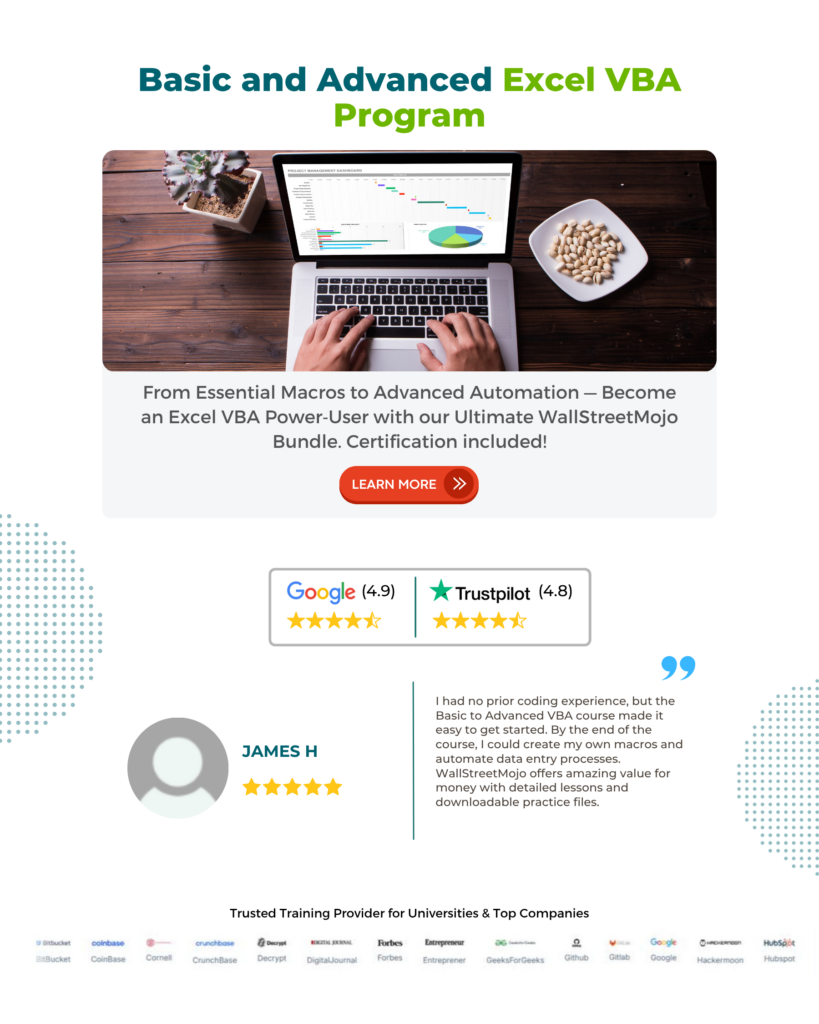
Frequently Asked Questions (FAQs)
• Activate the desired window or application.
• Simulate the keystrokes for saving, such as Ctrl+S.
• Use the SendKeys method to send the keystrokes.
• The application will process the keystrokes and save the file.
• Timing issues: The SendKeys method may execute before the target application is ready to receive the keystrokes.
• Focus issues: The active window may change during the execution, causing the keystrokes to be sent to the wrong application.
• Security settings: Some applications or security configurations may block or restrict the use of SendKeys for automation.
• Inconsistent behaviour: SendKeys can have varying results across different versions of Excel or operating systems.
• Alternative approaches: It’s recommended to use alternative methods, like the Excel object model or external libraries, for more reliable and controlled automation tasks.
• string: The required parameter specifying the keystrokes or combinations to send.
• Wait: An optional parameter indicating whether VBA should wait for the keystrokes to be processed by the active window.
SendKeys simulates keystrokes and can be used to automate tasks by sending keyboard input to the active window or application.
Click triggers a mouse click event and is typically used to interact with buttons, checkboxes, or other clickable elements in the user interface.
Recommended Articles
This has been a guide to VBA SendKeys. Here we explain how to use SendKeys method in excel VBA code, with examples & downloadable excel template. You can learn more from the following articles –
Leave a Reply