What is Active Cell in Excel VBA?
In Excel VBA, an active cell refers to a worksheet’s currently selected cell or range of cells. It is the cell that is currently highlighted or in focus, and any actions or operations performed in VBA will usually affect it.
VBA active cell value can be manipulated or referenced using the ActiveCell object. This object provides properties and methods to interact with the selected cell. For example, you can retrieve or set the active cell’s value, format it, or perform calculations based on its contents. Consider an example where the VBA active cell address is “A1”. Here, its value is “Sample String”. To print its value, we use the ActiveCell function present in Excel VBA using the code below.
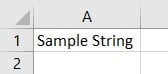
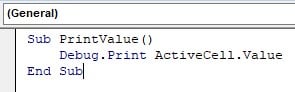
Since our cursor is clicked on cell “A1”, it is referenced using the ActiveCell.Value function. It will print the cell “A1” value in the Immediate tab as output.
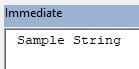
Similarly, ActiveCell can be used to perform the select value as shown below. It will overwrite the previously available value present in cell “A1,” as mentioned before. So now the value in cell A1 is as seen below:

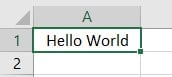
Table of contents
Key Takeaways
- The VBA Active Cell is specific to the active worksheet and refers to the currently active cell.
- We can reference the active cell value using the ActiveCell object.
- By referencing the elements using the ActiveCell object, we can perform operations and calculations on them.
- Be mindful of the performance when working with ActiveCell.
- ActiveCell can be referred to return various parameters such as, row number, column number, customizing font to name a few examples.
Excel VBA 5-in-1 Course Bundle – (30+ Hours of Expert-Led Training)
If you want to learn Excel and VBA professionally, then the Excel VBA 5-in-1 Course Bundle (30+ hours) is the perfect solution. Whether you’re a beginner or an experienced user, this bundle covers it all – from Basic Excel VBA to Event Automation, Error Handling, Email Automation, and real-world applications with downloadable templates.
Referencing in Excel VBA
In Excel VBA, referencing allows you to access and manipulate various elements in an Excel workbook, such as cells, ranges, worksheets, and workbooks. By referencing these elements, you can perform operations on them, retrieve their values, modify their properties, or apply calculations.
There are multiple ways to reference elements in Excel VBA.
#1 – Cell Reference
It is possible to reference a specific VBA active cell address by specifying its rows and columns.
Consider the following ActiveCell Value shown below:
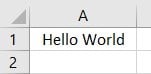
We can reference VBA active cell value by calling its address also. For example, both ways are shown below:

We can change the value of ActiveCell “A1” using Cells() in Excel VBA as shown:
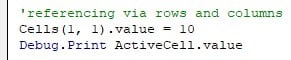
Code:
Sub CellRef()
‘address
Debug.Print Range(“A1”).Value
‘referencing via rows and columns
Cells(1, 1).Value = 10
Debug.Print ActiveCell.Value
End Sub
The output will print these values.
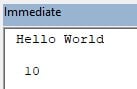
Also, the value in cell “A1” will be changed to 10.

#2 – Range Reference
It is possible to perform active cell select a range of cells in VBA Excel.
By address call:
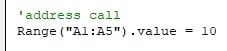
It will print 10 from cells A1-A5, as shown.

By row and column calls using the Cells function:

It will print 20 in cells C1-C3:
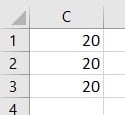
Code:
Sub RangeRef()
‘address call
Range(“A1:A5”).Value = 10
‘call by rows and columns
Range(Cells(1, 3), Cells(3, 3)).Value = 20
End Sub
#3 – Worksheet Reference
Cells can be referenced in a worksheet by their worksheet names or indices and cell addresses.
Consider the cell “A1” has the value:
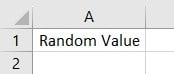
The values can be referenced by specifying the worksheet and cell address.

Or by calling the worksheet index.

It will result in the output of cell “A1” in both cases is printed in the Immediate tab.
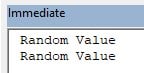
Code:
Sub WorksheetRef()
‘name call
Debug.Print Worksheets(“Sheet1”).Range(“A1”).Value
‘index call
Debug.Print Worksheets(1).Range(“A1”).Value
End Sub
#4 – Workbook Reference
The VBA Active Cell value can be referenced by calling the workbook we’re currently working in as shown. Let us suppose our workbook’s called Excel Format.
Consider the cell “A1” having value:
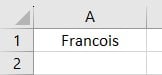
This value can be referenced by referencing the Workbook name, then the worksheet name, and then calling the address.

The workbook can also be opened and referred to using the file directory path of the Excel Workbook:

Which will print the output:
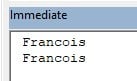
Note: Substitute the path of the Excel file with regards to the Excel file path present in your system.
Code:
Sub WorkbookRef()
‘name call
Debug.Print Workbooks(“ExcelFormat.xlsm”).Worksheets(“Sheet1”).Range(“A1”).Value
‘directory call
Debug.Print Workbooks.Open(“C:\Users\Ananth\Downloads\ExcelFormat.xlsm”).Worksheets(1).Range(“A1”).Value
End Sub
Active Cell Address, Value, Row and Column Number
To access the address, cell value, row number, and column number of the active cell in Excel VBA, you can use the properties of the ActiveCell object. Consider the cell value:

Shown below are the ways to Print the values mentioned earlier.
- VBA ACTIVE CELL ADDRESS:
It can be done by using the VBA Address property present in ActiveCell as shown below:

- VBA ACTIVE CELL VALUE:
It can be performed by printing the value of the Active Cell as shown below:

- VBA ACTIVE CELL ROW:
Using the Row attribute in ActiveCell, the Row number of the active cell can be printed as shown:

- VBA ACTIVE CELL COLUMN NUMBER:
By calling the Column attribute present in ActiveCell, the column number is printed as shown:

It will print the output of all four in the Immediate tab, as seen below:
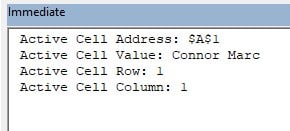
Code:
Sub ValueRef()
‘address ref
Debug.Print “Active Cell Address: ” & ActiveCell.Address
‘value ref
Debug.Print “Active Cell Value: ” & ActiveCell.Value
‘row ref
Debug.Print “Active Cell Row: ” & ActiveCell.Row
‘column number ref
Debug.Print “Active Cell Column: ” & ActiveCell.Column
End Sub
Parameters of Active Cell In Excel VBA
In Excel VBA, the ActiveCell object provides several properties that allow you to access and manipulate various aspects of the active cell. Consider the following example.
A function is defined in the Active Cell “A1,” as shown below. The value is currently 75.

There are several parameters from which the Active Cell can be referenced with:
- Address: ActiveCell can return the address of an active cell as shown:

- Value: ActiveCell returns the value of the given active cell:

- Row: ActiveCell can print the row number of the currently active cell:

- Column: ActiveCell returns the value of the column number of the VBA active cell:

- Formula: ActiveCell can print the Excel formula, if any, that is used in the active cell. If there is no formula used, it will simply print the value:

- Font: ActiveCell can customize fonts by changing font size, font colour to name a few examples:

Here, we’ve made the value bold and changed the font size to 12 and also changed the font colour to blue using VBA RGB(0,0,255).
Code:
Sub ParamRef()
‘address ref
Debug.Print “Active Cell Address: ” & ActiveCell.Address
‘value ref
Debug.Print “Active Cell Value: ” & ActiveCell.Value
‘row ref
Debug.Print “Active Cell Row: ” & ActiveCell.Row
‘column number ref
Debug.Print “Active Cell Column: ” & ActiveCell.Column
‘formula ref
Debug.Print “Active Cell Formula: ” & ActiveCell.Formula
‘FONT
ActiveCell.Font.Bold = True
ActiveCell.Font.Size = 12
ActiveCell.Font.Color = RGB(0, 0, 255)
End Sub
It will print the output:
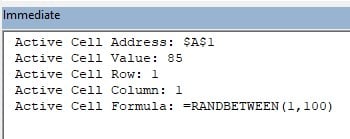
Important Things To Note
- Don’t assume the active cell remains unchanged: Remember that the active cell can change as users interact with the worksheet. If your code relies on a specific active cell, consider capturing the initial selection or using event triggers to respond to specific user actions.
- Don’t overuse selecting or activating cells: Minimize using the Select and Activate methods, as they can slow down code execution and make it harder to maintain. Instead, directly reference cells and ranges without activating or selecting them.
- Do check the worksheet context: Ensure that the active cell is within the intended worksheet. Activate the desired worksheet explicitly using the Activate method or reference the cell using a specific worksheet object to avoid unintentionally manipulating the wrong worksheet.
- Do validate the active cell contents: Before performing actions based on the active cell’s value, perform appropriate data validation and error handling. Check for the expected VBA data types, handle potential errors, and account for empty or invalid cell values.
- Do store the active cell in a variable: If you plan to reference the active cell multiple times in your code, it’s a good practice to store it in a variable. It can help improve code readability and performance.
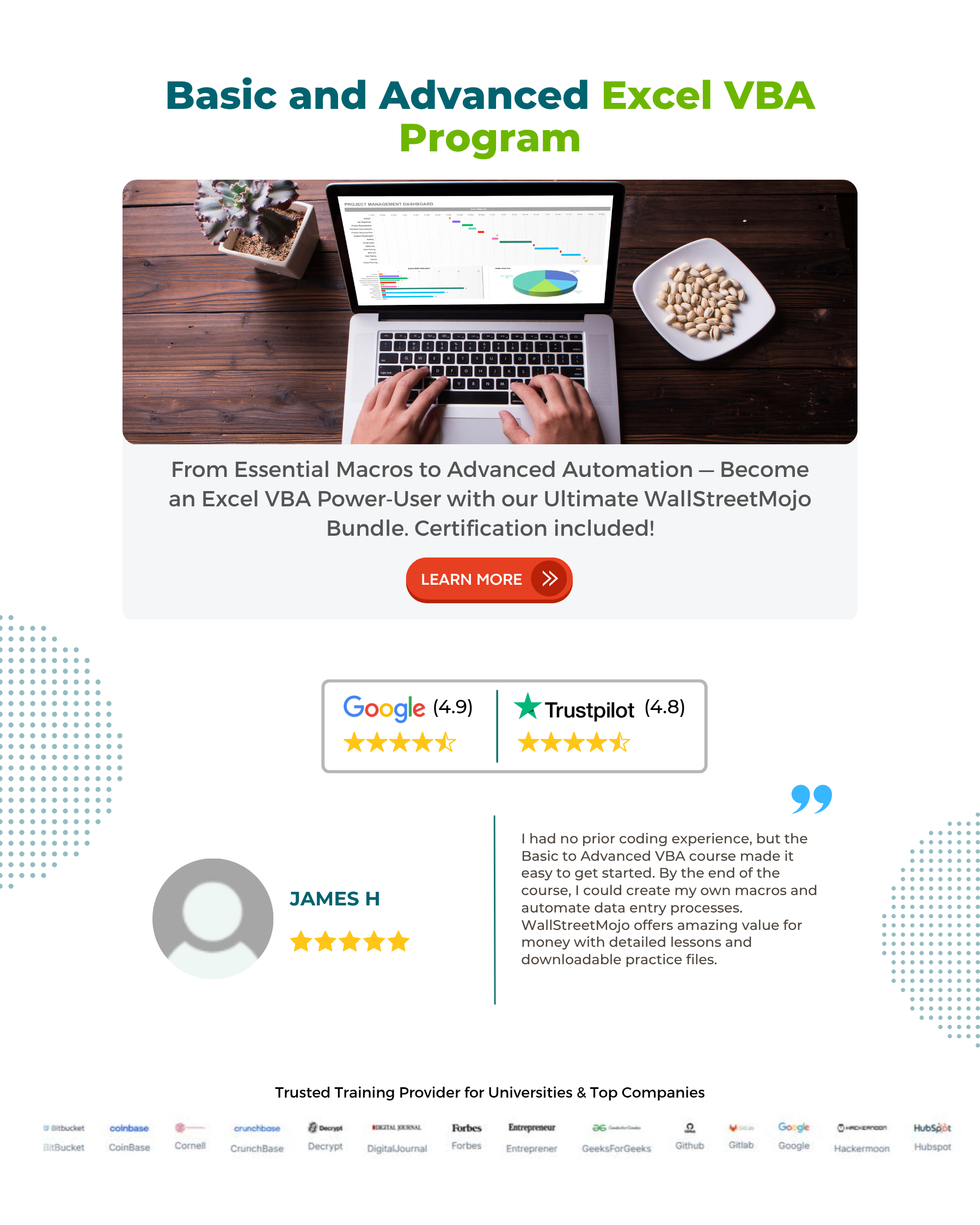
Frequently Asked Questions (FAQs)
To activate a cell range in Excel VBA:
• Define the range using a variable or directly reference it.
• Use the Activate method on the desired cell within the range to make it the active cell.
• No cell is currently selected.
• The code is not properly referencing the ActiveCell object.
• The ActiveCell property is being used in the wrong context (e.g., referencing a different worksheet).
• The ActiveCell object is being overwritten or modified by other code before it can be accessed.
• Use the SpecialCells method on the range object to identify all cells with a specific attribute, such as being visible, having a value, or containing formulas.
• Specify the attribute using the xlCellTypeVisible constant, xlCellTypeConstants constant, or xlCellTypeFormulas constant, depending on your selection criteria.
• Assign the result to a range variable.
• Use the Select method on the range variable to select all the identified cells.
Recommended Articles
This has been a guide to VBA Active Cell. Here we look in multiple ways and parameters to reference elements in Excel VBA with examples. You can learn more from the following articles –
Leave a Reply