What is VBA Code to Send Emails from Excel?
VBA code provides a straightforward and efficient way to send emails directly from Excel. Utilizing the Outlook application can automate composing and sending emails, saving time and streamlining your communication tasks.
By leveraging VBA code to send emails from Excel, you can automate repetitive tasks, improve efficiency, and ensure accurate and timely communication with your recipients. It provides seamless integration between Excel and Outlook, offering a convenient solution for email automation.
In the provided screenshot, we observe that upon running the VBA code to send emails, Outlook is launched automatically.
The email’s recipients, subject line, and body are populated per the code, streamlining the process and offering a time-saving solution for sending bulk emails.
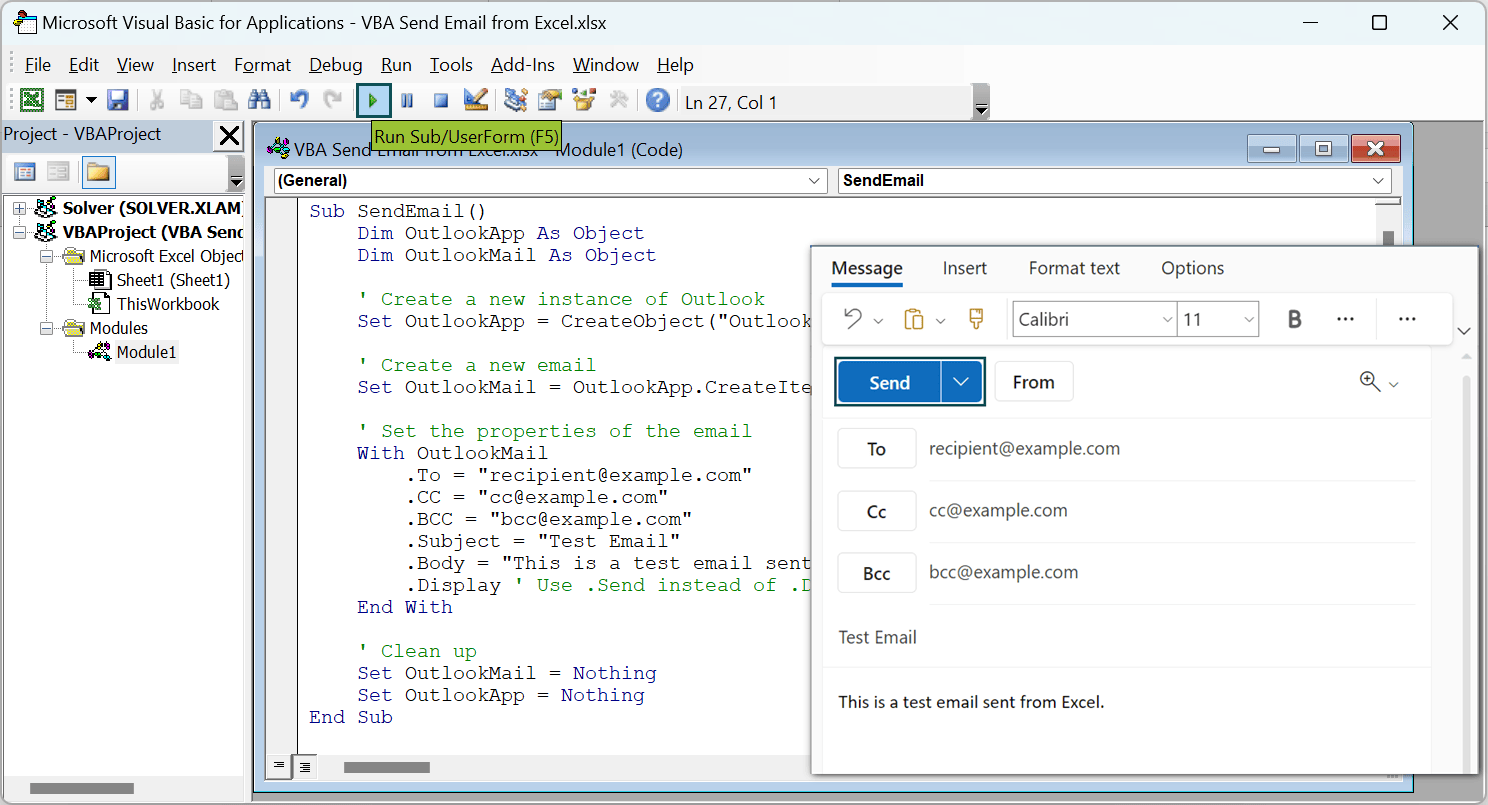
Table of contents
Key Takeaways
- VBA send Email from Excel enables you to automate the process of sending emails from Excel, allowing you to efficiently communicate with multiple recipients.
- To send emails from Excel using VBA, you need to enable Outlook scripting and set a reference to the Microsoft Outlook Object Library.
- The key steps in VBA send Email from Excel include creating a new instance of Outlook, creating a new email item, setting the email properties such as recipients, subject, and body, and finally sending the email using the “.Send” method.
- VBA send email provides flexibility in managing email properties, such as specifying recipients in the To, CC, and BCC fields, setting the subject and body of the email, and even attaching files to the email using the Attachments property.
Excel VBA 5-in-1 Course Bundle – (30+ Hours of Expert-Led Training)
If you want to learn Excel and VBA professionally, then the Excel VBA 5-in-1 Course Bundle (30+ hours) is the perfect solution. Whether you’re a beginner or an experienced user, this bundle covers it all – from Basic Excel VBA to Event Automation, Error Handling, Email Automation, and real-world applications with downloadable templates.
How to Send Emails from Excel using VBA?
To send emails from Excel using VBA, follow the below steps.
- You must initialize variables to store important information, such as the Outlook application object, mail item object, email subject, body, and recipient’s email address.
- Then, create an instance of the Outlook application and a new mail item object.
- By setting its relevant properties, such as subject, body, and recipients, you can customize the email’s content.
- Additionally, you can attach files to the email using the “Attachments.Add” method.
How to Set Reference to Microsoft Office Library?
Setting a reference to the Microsoft Office Library is an essential step when working with VBA code in Excel to utilize the features of Microsoft Office applications like Outlook. This reference allows us to access the necessary objects and methods provided by the library to interact with Outlook seamlessly. Here’s a step-by-step guide on how to set the reference:
- Open the Visual Basic Editor (VBE) in Excel by pressing Alt+F11.
- In the VBE window, go to the “Tools” menu and select “References” from the dropdown menu. It will open the References dialog box.
- In the References dialog box, scroll down, and locate the entry named “Microsoft Office XX.0 Object Library,” where “XX.0” represents the version number of Microsoft Office installed on your computer. For example, it could be “Microsoft Office 16.0 Object Library” for Office 2016.
- Check the checkbox next to the “Microsoft Office XX.0 Object Library” entry to select it.
- Click the “OK” button to close the References dialog box.
By setting this reference, you enable VBA to recognize and use the objects and methods available in the Microsoft Office Library, including those specific to Outlook. It allows you to write VBA code that interacts with Outlook, such as sending emails or manipulating Outlook items.
Setting the reference to the Microsoft Office Library ensures that your VBA code can access the necessary tools and functions required to integrate Excel with Outlook seamlessly, opening a wide range of possibilities for automating tasks and enhancing productivity.
13 Easy Steps to Send Emails from Excel
To send emails using VBA, you need to utilize the capabilities of Microsoft Outlook. Here’s an example of a VBA code that sends an email from Excel using Outlook. Please refer to the “VBA Send Email from Excel” Excel workbook.
- Step 1: Open the Visual Basic Editor (VBE) by pressing the Alt + F11 keys in Excel or clicking on the Developer tab in the ribbon and then click on the Visual Basic button. In the VBE, click on the Insert menu and select Module to create a new module.
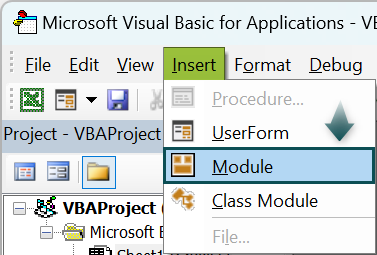
- Step 2: In the new module, start the sub-procedure named “SendEmail” and declare the necessary variables and objects.
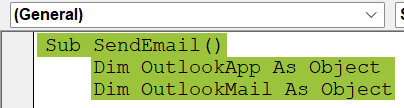
For this example, we declare two variables (“OutlookApp” and “OutlookMail”) as objects. These objects will be used to interact with Outlook and create an email.
- Step 3: Create a new instance of Outlook.

The above line of code creates a new instance of Outlook and assigns it to the “OutlookApp” variable. It allows us to access Outlook’s features and functionality.
- Step 4: Create a new email using the Set statement.

This line of code creates a new email item using the “CreateItem” method of the “OutlookApp” object. The argument “(0)” specifies that we want to create an email.
- Step 5: Set the properties of the email.

This code sets various properties of the email using the “With” statement.
- Step 6: Enter the recipient’s email address.

Replace “recipient@example.com” with the actual email address of the recipient. This sets the “To” field of the email.
- Step 7: Enter any additional recipients in the CC field.

If you want to carbon copy (CC) anyone on the email, replace “cc@example.com” with the actual email address. It sets the “CC” field of the email.
- Step 8: Enter any additional recipients in the BCC field.

If you want to blind carbon copy (BCC) anyone on the email, replace “bcc@example.com” with the actual email address. It sets the “BCC” field of the email.
- Step 9: Set the subject of the email.

Replace “Test Email” with the desired subject for your email. It sets the subject field of the email.
- Step 10: Set the body of the email.

Replace the text within the quotation marks with the desired content for the email body. It sets the body text of the email.
- Step 11: Display the email for review before sending.

This line of code displays the email in Outlook, allowing you to review the content, recipients, subject, and any other details before sending it.
If you want to send the email, just replace “.Display” with “.Send” in the VBA code.
- Step 12: Clean up the Outlook objects.
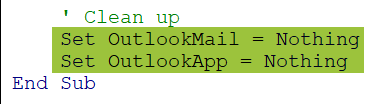
After sending the email or closing the email window, it’s essential to release the Outlook objects from memory to free up system resources. Setting the objects to Nothing ensures proper cleanup.
- Step 13: Customize and test the code.
Explanation: Customize the code by modifying the email recipients, subject, body, and any other properties to suit your specific needs. Once you’re done, run the code to send the email from Excel using VBA.
Here is the full code:
Sub SendEmail()
Dim OutlookApp As Object
Dim OutlookMail As Object
' Create a new instance of Outlook
Set OutlookApp = CreateObject("Outlook.Application")
' Create a new email
Set OutlookMail = OutlookApp.CreateItem(0)
' Set the properties of the email
With OutlookMail
.To = "recipient@example.com"
.CC = "cc@example.com"
.BCC = "bcc@example.com"
.Subject = "Test Email"
.Body = "This is a test email sent from Excel."
.Display ' Use .Send instead of .Display to send the email without displaying it
End With
' Clean up
Set OutlookMail = Nothing
Set OutlookApp = Nothing
End Sub
Important Things to Note
While sending emails from Excel using VBA, there are a few vital considerations to keep in mind.
- When using VBA to send an email from Excel with a signature, the signature might not be included in the email body. By default, the email body generated through VBA does not include the user’s Outlook signature. Therefore, you need to handle the signature explicitly in your VBA code.
- When using VBA send email from Excel range, specify the range of cells containing the email data accurately. Ensure that the range includes all the necessary information, including recipients’ email addresses, subject, and body content. Ensure the data is organized to align with the desired email layout.
- When using VBA send email from Excel using the Oft template, it’s important to declare and create the necessary Outlook objects, such as “Outlook.Application” and “Outlook.MailItem”.
- When using VBA send email from Excel with attachment, ensure that the file paths are accurate and accessible. Consider the file size limitations and the impact on email delivery and recipient’s inbox.
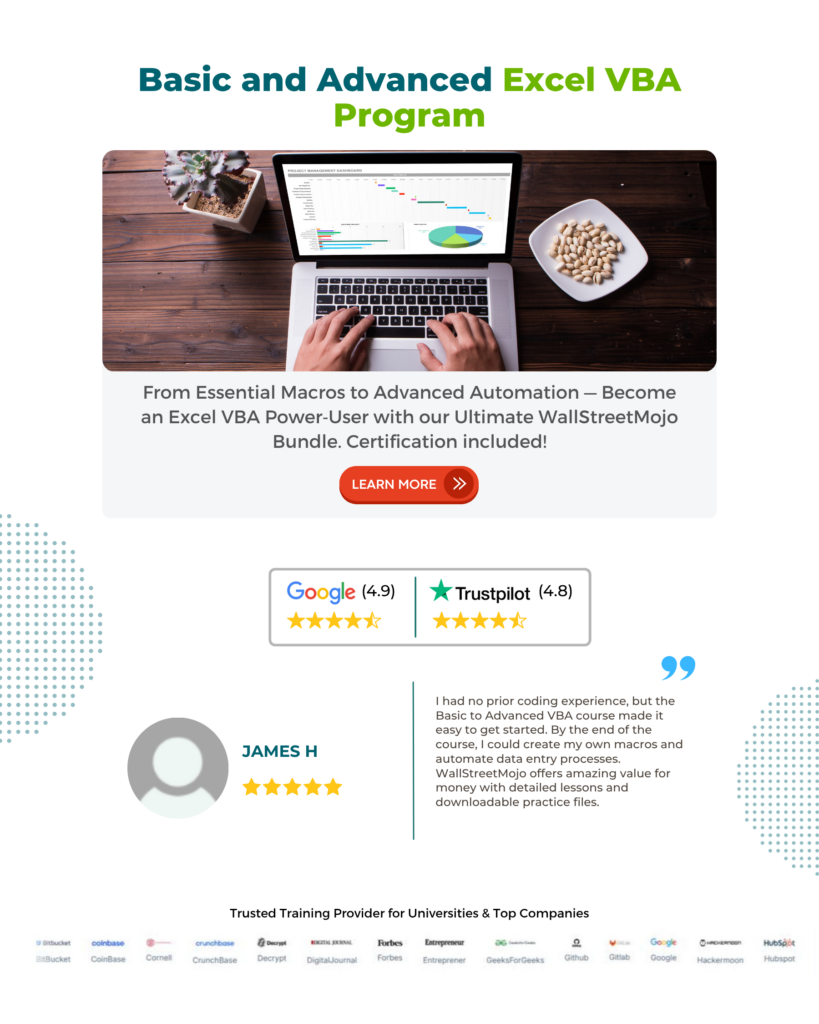
Frequently Asked Questions (FAQs)
To send bulk emails from Outlook using VBA Send email from Excel, follow these steps:
1) First, prepare an Excel spreadsheet with recipient email addresses, subject, and body.
2) Enable the “Microsoft Outlook Object Library” reference in VBA.
3) Write a VBA subroutine to loop through the spreadsheet and send emails. To do this, you can set a range of email addresses in the worksheet using the following code:
“Set Recipients = Sheets(“Sheet1”).Range(“A2:A10”) ‘ Update the sheet name and range as needed”
4) Declare Outlook application and mail item objects using “CreateObject”.
5) Iterate through each recipient using a loop. This can be done using the following code:
For Each Recipient In Recipients
‘ Create a new email
Set OutlookMail = OutlookApp.CreateItem(0)
6) Run the code to send bulk emails.
7) Review and refine the process based on feedback.
To automatically send emails from an Excel worksheet using VBA, follow these steps:
1) Prepare the Excel worksheet with the necessary email details.
2) Enable the “Microsoft Outlook Object Library” reference in VBA.
3) Write a VBA subroutine to send emails automatically.
4) Declare Outlook application and mail item objects using “CreateObject”.
5) Set the mail item properties (To, CC, BCC, Subject, Body) using worksheet values.
6) Use the “Send” method to send emails without user intervention.
7) Implement error handling to handle potential issues.
8) Test and debug the code for accuracy.
9) Add attachments or HTML formatting if needed.
10) Run the VBA code to send emails automatically.
11) Verify successful delivery in the recipient’s inbox.
12) Refine and optimize the code based on feedback.
13) Maintain a backup of the Excel file with the VBA code.
After creating a new mail item and setting the basic properties like recipients, subject, and body, you can attach a file to the email. Specify the file path of the attachment and use the “Attachments.Add” method to include the file.
For example, to attach a file named “example.xlsx” located in the same directory as the Excel file, you would use the following code:
EmailItem.Attachments.Add ThisWorkbook.Path & “\example.xlsx”
Now, send the email using the “Send” method: EmailItem.Send
There could be several reasons why sending emails from Excel VBA is not working. Here are some possible causes:
1) Incorrect email configuration: Ensure that the email account settings, including the SMTP server, port, username, and password, are correctly configured in your VBA code.
2) Missing or outdated references: Check if the required references, such as Microsoft Outlook or Microsoft Office Object Library, are properly set in the VBA editor.
3) Security settings or firewalls: Antivirus software, firewalls, or security settings on your computer may be blocking the email-sending functionality.
4) Invalid email addresses: Verify that the email addresses of the recipients, CC, or BCC are entered correctly. Internal restrictions: Some organizations may have internal policies or restrictions that prevent automated emails from being sent.
Recommended Articles
This has been a guide to VBA Send Email from Excel. Here we explain how to send emails from excel using VBA code in 13 easy steps. You can learn more from the following articles –
Leave a Reply